使用PowerShell将字符串拆分为数组
In this article, I am going to explain the PowerShell script to split a string into an array. Before I explain it, let me just give you a small introduction of the PowerShell and the PowerShell array.
在本文中,我将解释PowerShell脚本将字符串拆分为数组。 在解释它之前,让我先简要介绍一下PowerShell和PowerShell数组。
什么是Microsoft PowerShell? (What is Microsoft PowerShell?)
PowerShell is a command-line language provided by Microsoft. It is the replacement of the Windows batch scripting tool known as DOS. It is mainly designed for the IT administrators to automate and control the Windows operating systems and other applications.
PowerShell是Microsoft提供的一种命令行语言。 它替代了Windows批处理脚本工具DOS。 它主要是为IT管理员设计的,用于自动化和控制Windows操作系统和其他应用程序。
Following are the benefits of the PowerShell:
以下是PowerShell的优点:
- It has a simple way to manipulate the operating system components and configurations 它具有操作操作系统组件和配置的简单方法
- It is more secure than running VB and other application Scripts 它比运行VB和其他应用程序脚本更安全。
- PowerShell allows complete access to the Microsoft.Net framework methods PowerShell允许完全访问Microsoft.Net框架方法
PowerShell中的数组 (Arrays in PowerShell)
Arrays are the fixed-sized, sequentially ordered collection of the elements of any data types. It can be considered as the collection of the operators or variables. The arrays within the PowerShell can be processed using FOR loop and WHILE loop because all the elements in the array are of the same type, and the size of the array is known. The array in the PowerShell can be declared as follows:
数组是任何数据类型的元素的固定大小,顺序排序的集合。 可以将其视为运算符或变量的集合。 可以使用FOR循环和WHILE循环来处理PowerShell中的数组,因为数组中的所有元素都是相同的类型,并且数组的大小是已知的。 PowerShell中的数组可以声明如下:
Array Syntax:
数组语法:
$Array=”Nisarg”, “Nirali”,”Dixit”,”Bharti”
$Array
Output:
输出:
Nisarg
Nirali
Dixit
Bharti
尼萨尔
尼拉里
迪克西特
巴蒂
See the following output:
请参阅以下输出:

In this article, I am going to explain a few methods, as well as the PowerShell string functions that are used to convert the string into an array. I have used the following functions of PowerShell.
在本文中,我将解释一些方法,以及用于将字符串转换为数组的PowerShell字符串函数。 我已经使用了PowerShell的以下功能。
- .ToCharArray() .ToCharArray()
- .split() 。分裂()
.TocharArray()函数 (The .TocharArray() function)
The .TocharArray() function copies the characters of the string into the Unicode character array. .As I mentioned above, PowerShell can access all types of Microsoft.Net framework; hence we can use this function within the PowerShell script. Now, for example, we want to convert the string into the array of the word.
.TocharArray()函数将字符串的字符复制到Unicode字符数组中。 如上文所述,PowerShell可以访问所有类型的Microsoft.Net框架; 因此我们可以在PowerShell脚本中使用此功能。 现在,例如,我们想将字符串转换为单词的数组。
Convert Input String in Char Array:
转换Char数组中的输入字符串:
$Inputstring ="SQL Server"
$CharArray =$InputString.ToCharArray()
$CharArray
Output
输出量
S
Q
L
S
e
r
v
e
r
小号
问
大号
小号
Ë
[R
v
Ë
[R
Following is the output of the script
以下是脚本的输出

As you can see, we store the input string in $Inputstring variable, convert the string into the character array by using.TocharArray() function and store it in $CharArray variable and print each character within the array.
如您所见,我们将输入字符串存储在$ Inputstring变量中,使用.TocharArray()函数将字符串转换为字符数组,然后将其存储在$ CharArray变量中,并打印数组中的每个字符。
.Split()函数 (The .Split() function)
The .Split() function splits the input string into the multiple substrings based on the delimiters, and it returns the array, and the array contains each element of the input string. By default, the function splits the string based on the whitespace characters like space, tabs, and line-breaks. If you want to split the string based on the specific character, then you must specify the character in the second argument. Following is the syntax of the .split() function.
的。 Split()函数根据定界符将输入字符串分成多个子字符串,并返回数组,该数组包含输入字符串的每个元素。 默认情况下,该函数根据空格字符(例如空格,制表符和换行符)分割字符串。 如果要根据特定字符分割字符串,则必须在第二个参数中指定字符。 以下是.split()函数的语法。
For example, we want to split the following string into an array of multiple words by “-” character. Following is the code:
例如,我们想用“-”字符将以下字符串分成多个单词的数组。 以下是代码:
Convert the input string into the array of the words:
将输入字符串转换为单词数组:
$Inputstring ="Microsoft-SQL-Server"
$CharArray =$InputString.Split("-")
$CharArray
Output:
输出:
Microsoft
SQL
Server
微软
SQL
服务器
Following is the output of the script:
以下是脚本的输出:

The above code splits the string based on the “-” character and saves the output in the $CharArray variable.
上面的代码基于“-”字符分割字符串,并将输出保存在$ CharArray变量中。
例子1 (Example 1)
If you want to access the specific word or the character in the output array, you must use the index of the array. Please note that the index of the array starts from 0. For example, in “Sonali Bhatt is Database Administrator” string, you want to print only “Sonali” and “Administrator” words. The index of the word “Sonali” within the array is zero, and the index of the word “Administrator” is four. The code should be written in the following way.
如果要访问输出数组中的特定单词或字符,则必须使用数组的索引。 请注意,数组的索引从0开始。例如,在“ Sonali Bhatt is Database Administrator ”字符串中,您只想打印“ Sonali ”和“ Administrator ”字样。 数组中单词“ Sonali ”的索引为零,单词“ 管理员 ”的索引为4。 该代码应以以下方式编写。
PowerShell Script:
PowerShell脚本:
$Inputstring ="Sonali Bhatt is Database Administrator"
$CharArray =$InputString.Split(" ")
$CharArray[0]
$CharArray[4]
Output:
输出:
Sonali
Administrator
索纳利
管理员
Following is the output:
以下是输出:

As you can see, we have assigned “Sonali Bhatt is a Database Administrator” string to $insputString variable, used .Split() function to split the string in multiple words and used index of the array to print the “Sonali” and “Administrator.”
如您所见,我们为$ insputString变量分配了“ Sonali Bhatt is a Database Administrator ”字符串,使用.Split()函数将字符串拆分为多个单词,并使用数组的索引打印“ Sonali ”和“ Administrator 。”
This method can be used to split the string into two separate variables. For example, if you want to save the word “Database” in the $String1 variable and “Administrator” in the $String2 variable, then you should write the code in the following way.
此方法可用于将字符串分成两个单独的变量。 例如,如果你想保存在$ String2的变量在$ String1中变量单词“ 数据库 ”和“ 管理员 ”,那么你应该写在下面的方法的代码。
PowerShell Script:
PowerShell脚本:
$Inputstring ="Sonali Bhatt is Database Administrator"
$CharArray =$InputString.Split(" ")
$String1= $CharArray[0]
$String2= $CharArray[4]
write-host $String1
write-host $String2
Output:
输出:
Sonali
Administrator
索纳利
管理员
See the following image:
见下图:
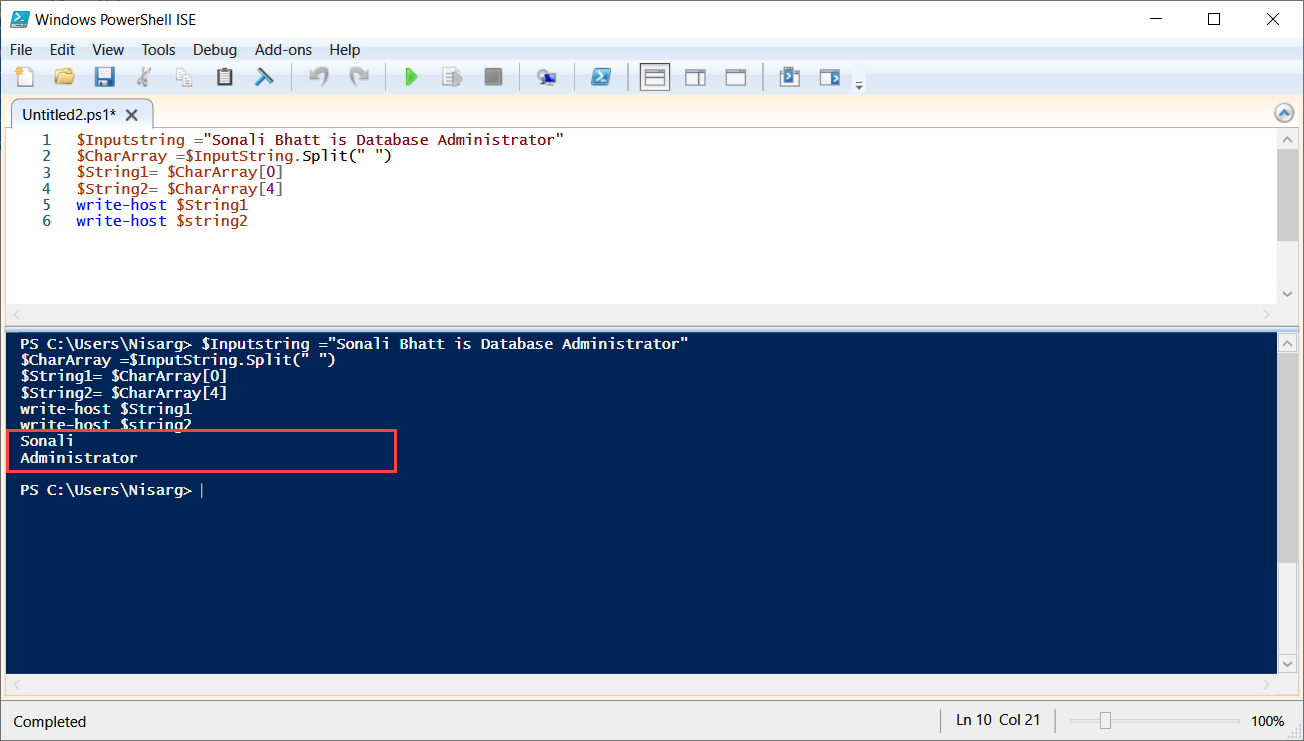
例子2 (Example 2)
If you want to convert the string into an array of the multiple substrings or words using the .split() function, then you must specify the additional argument named “number of the substring.” For example, we want to convert the string ”Nisarg, Nirali and Sonali are Database Administrators” in the array of the three substrings, and the entire string must be split with “,” delimiter then the code should be written in the following way:
如果要使用.split()函数将字符串转换为多个子字符串或单词的数组,则必须指定名为“ 子字符串编号 ”的附加参数。 例如,我们要在三个子字符串的数组中转换字符串“ Nisarg,Nirali和Sonali是Database Administrators ”,并且必须用“,”定界符来分隔整个字符串,然后应按以下方式编写代码:
PowerShell Script:
PowerShell脚本:
$string = "Nisarg,Nirali,Sonali are Database Administrators"
$array= $string.Split(",")
$array[0]
$array[1]
$array[2]
Output:
输出:
Nisarg Nirali Sonali are Database Administrators
Nisarg Nirali Sonali是数据库管理员
Following is the output:
以下是输出:

例子3 (Example 3)
If you want to convert the string into an array of the multiple characters based on the arbitrary characters, then you must specify “\d” OR “\D”. If you specify “\d”, then it returns the array of the characters, and if you specify “\D” then, it returns the array of the numbers. For example, if we want to extract the “1987” from the “b1i9r8t7h” input string, then the code should be written as follows:
如果要将字符串转换为基于任意字符的多个字符组成的数组,则必须指定“ \ d ”或“ \ D ”。 如果指定“ \ d ”,则返回字符数组,如果指定“ \ D”,则返回数字数组。 例如,如果我们要从“ b1i9r8t7h ”输入字符串中提取“ 1987 ”,则代码应编写如下:
PowerShell Script:
PowerShell脚本:
$string = "b1i9r8t7h"
$array= $string -split "\D"
$array
Output:
输出:
1
9
8
7
1个
9
8
7
Following is the output:
以下是输出:

If you want to extract “birth” then the code should be written as following:
如果要提取“出生”,则代码应编写如下:
PowerShell Script:
PowerShell脚本:
$string = "b1i9r8t7h"
$array= $string -split "\d"
$array
Output:
输出:
b
i
r
t
h
b
一世
[R
Ť
H
Following is the output:
以下是输出:

摘要 (Summary)
In this article, I have explained the PowerShell scripting and array variable in PowerShell. Additionally, I also covered PowerShell scripts to split a string into an array using .toCharArray() and .split() functions.
在本文中,我已经解释了PowerShell中的PowerShell脚本和数组变量。 此外,我还介绍了PowerShell脚本,这些脚本使用.toCharArray()和.split()函数将字符串拆分为数组。
翻译自: https://www.sqlshack.com/powershell-split-a-string-into-an-array/
使用PowerShell将字符串拆分为数组相关推荐
- 在Bash中将字符串拆分为数组
本文翻译自:Split string into an array in Bash In a Bash script I would like to split a line into pieces a ...
- 数据库拆分字符串函数_PHP | 不使用库函数将逗号分隔的字符串拆分为数组
数据库拆分字符串函数 Given a string with comma delimited, we have to split it into an array. 给定一个以逗号分隔的字符串,我们必 ...
- Java 将字符串拆分成数组,实现字符串组合
将字符串拆分成数组和实现字符串组合 不能使用语言的基本分割组合函数(如 Java 的 String.split, php 的 explode 和 implode) 1) 字符串拆分成字符串数组,如&q ...
- 把一个字符串分割成数组 php_PHP怎么将字符串拆分成数组
在日常项目开发过程中,较长的字符串可能需要被拆分成数组形式,以便被展现或用于判断验证.那么将字符串拆分成数组,也很容易实现,我们可以直接通过PHP中的explode函数来进行拆分. 下面我们就通过简单 ...
- java字符串拆分成数组_Java StringUtils字符串分割转数组的实现
Java StringUtils字符串分割转数组的实现 发布于 2020-6-7| 复制链接 摘记: 我们在日常开发时会经常遇到将一个字符串按照指定的字符进行分割.这时,我们往往会想到使用str.sp ...
- split字符串拆分踩坑记录(字符串拆分后数组下标越界解决方案)
问题:使用split拆分String字符串后拿到的数组发现一个问题-如果拆分的字符串根据拆分规则拆分后,后面的位置上如果没有值,则split返回的数组会自动忽略它们. demo:代码第四行返回的数组长 ...
- Java与JavaScript 完美实现字符串拆分(利用数组存储)与合并的互逆操作
Java: String typeStr = "1,2"; String[] typeArray = typeStr.split(","); typeStr = ...
- java字符串拆分成数组_用Java实现JVM第八章《数组和字符串》
小傅哥 | https://bugstack.cn 沉淀.分享.成长,专注于原创专题案例,以最易学习编程的方式分享知识,让自己和他人都能有所收获.目前已完成的专题有:Netty4.x实战专题案例.用J ...
- 在Python中将字符串拆分为字符数组
Given a string and we have to split into array of characters in Python. 给定一个字符串,我们必须在Python中拆分为字符数组. ...
最新文章
- 高性能ASP.NET系统架构设计
- 有没有python与机械结合的工作-用 Python 自动化办公,我与大神之间的差距一下就...
- Ollivander's Inventory(连接查询、单表双实例、子查询)
- Data Guard相关参数学习介绍
- poj 3104 Drying(二分查找)
- python不定长参数详解
- matlab设置固定的窗宽窗位,python实现CT窗宽窗位的调整(即指定HU值保存图像)...
- ubuntu1204 dvd 用tweak后界面起不来 swap设置4g足够32位系统软件用
- 关于数据结构,这个重要概念不了解可不行
- python json转换与处理
- android音量知识总结
- 包装模式就是这么简单啦
- JVM内存与垃圾回收篇
- 小米miui adb删除自带软件
- 2个抖音工程师搞出新工具,意外风靡字节内部,项目经理用上安静多了,程序员不骗程序员...
- Day 7 输出m到n之间的素数
- 防水测试设备的应用领域
- sqoop -D 指定资源池( mapred.job.queue.name=root.myqueue)或者( mapred.job.queuename=root.myqueue)
- reference other engineer's code to explain wheather linux terminal can display matplotlib' figure
- excel网页服务器端,Excel服务VI――用Excel Web Services创建应用程
热门文章
- object转成实体对象_面向对象的TypeScript-序列化与反序列化(1)
- idea重写接口没有@override_接口和抽象有什么区别?
- list 和 iterate
- firstchild.data与childNodes[0].nodeValue意思(转)
- x+=y与x=x+y有什么区别?
- Silverlight中需要用到模板选择器(DataTemplateSelector)的替代方案
- 《算法导论》CLRS算法C++实现(六)P100 基数排序
- form input类型
- WebLogic Clustering Overview Slides
- 时间的正则表达式验证