关于PVS-Studio如何在用于... PVS-Studio的库中发现错误的故事
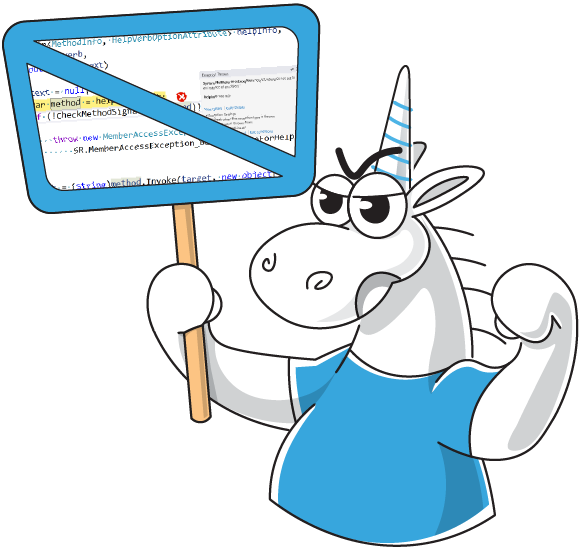
This is a short story about how PVS-Studio helped us find an error in the source code of the library used in PVS-Studio. And it was not a theoretical error but an actual one — the error appeared in practice when using the library in the analyzer.
这是关于PVS-Studio如何帮助我们在PVS-Studio中使用的库的源代码中发现错误的简短故事。 这不是理论错误,而是实际错误–在分析仪中使用该库时,实际上会出现该错误。
In PVS-Studio_Cmd (as well as some other utilities) we use a special library for parsing command line arguments — CommandLine.
在PVS-Studio_Cmd(以及其他一些实用程序)中,我们使用一个特殊的库来解析命令行参数-CommandLine。
Today I supported the new mode in PVS-Studio_Cmd and it so happened that I had to use this library for parsing command line arguments. While writing the code, I also debug it because I have to work with unfamiliar APIs.
今天,我在PVS-Studio_Cmd中支持新模式,碰巧我不得不使用该库来解析命令行参数。 在编写代码时,我还调试了它,因为我必须使用不熟悉的API。
So, the code is written, compiled, executed and...
因此,代码被编写,编译,执行并...

Code execution goes inside the library where an exception of the NullReferenceException type occurs. It's not so clear from the side — I don't pass any null references into the method.
代码执行在发生NullReferenceException类型的异常的库中进行。 从侧面看还不太清楚-我没有将任何null引用传递给该方法。
To be sure, I look at comments to the callee method. It is hardly probable that they describe the conditions of occurrence of an exception of the NullReferenceException type (as it seems to me usually exceptions of this type are not provided for).
可以肯定的是,我查看对被调用方方法的注释。 它们几乎不可能描述NullReferenceException类型的异常的发生条件(在我看来,通常不提供这种类型的异常)。

There is no information about NullReferenceException in the comments to the method (which, however, is expected).
在方法的注释中没有有关NullReferenceException的信息(但是,这是预期的)。
To see what exactly causes the exception (and where it occurs), I decided to download the project's source code, build it and add a reference to the debug version of the library to the analyzer. The source code of the project is available at GitHub. We need the version 1.9.71 of the library. It is the one used in the analyzer now.
为了查看导致异常的确切原因(及其发生的位置),我决定下载该项目的源代码,进行构建,然后将对库的调试版本的引用添加到分析器。 该项目的源代码可从GitHub获得 。 我们需要该库的1.9.71版本。 现在是分析仪中使用的一种。
I download the corresponding version of the source code, build the library, add a reference to the debug library to the analyzer, execute the code and see:
我下载了相应版本的源代码,构建了库,将对调试库的引用添加到了分析器,执行了代码并看到:

So, the place where the exception occurs is clear — helpInfo has a null value, which causes an exception of the NullReferenceException type when accessing the Left property.
因此,发生异常的地方很清楚— helpInfo具有null值,当访问Left属性时,它将导致NullReferenceException类型的异常。
I started thinking about it. Recently, PVS-Studio for C# has been well improved in various aspects, including the search for dereferencing of potentially null references. In particular, the interprocedural analysis was improved in a number of ways. That's why I was immediately interested in checking the source code to understand if PVS-Studio could find the error under discussion.
我开始考虑它。 最近,用于C#的PVS-Studio在各个方面都得到了很好的改进,包括对可能为空的引用进行解引用的搜索。 特别是,过程间分析在许多方面得到了改进。 这就是为什么我立即对检查源代码感兴趣,以了解PVS-Studio是否可以找到正在讨论的错误。
I checked the source code and among other warnings I saw exactly what I hoped for.
我检查了源代码,并在其他警告中看到了我所希望的。
PVS-Studio警告 (PVS-Studio warning)
:
:
V3080 Possible null dereference inside method at 'helpInfo.Left'. Consider inspecting the 2nd argument: helpInfo. Parser.cs 405V3080在'helpInfo.Left'的方法内部可能存在空取消引用。 考虑检查第二个参数:helpInfo。 解析器405
Yeah, this is it! That's exactly what we need. Let's take a more detailed look at the source code.
是的,就是这样! 这正是我们所需要的。 让我们更详细地看一下源代码。
private bool DoParseArgumentsVerbs(string[] args, object options, ref object verbInstance)
{var verbs = ReflectionHelper.RetrievePropertyList<VerbOptionAttribute>(options);var helpInfo = ReflectionHelper.RetrieveMethod<HelpVerbOptionAttribute>(options);if (args.Length == 0){if (helpInfo != null || _settings.HelpWriter != null){DisplayHelpVerbText(options, helpInfo, null); // <=}return false;}....
}
The analyzer issues a warning for calling the DisplayHelpVerbText method and warns about the second argument — helpInfo. Pay attention that this method is located in the then-branch of the if statement. The conditional expression is composed in such a way that the then-branch can be executed at the next values of the variables:
分析器会发出警告,提示调用DisplayHelpVerbText方法并警告第二个参数helpInfo 。 请注意,此方法位于if语句的then -branch中。 条件表达式的编写方式使得可以在变量的下一个值处执行then -branch:
helpInfo == null;
helpInfo == null ;
_settings.HelpWriter != null;
_settings.HelpWriter!= null ;
Let's see the body of the DisplayHelpVerbText method:
让我们看一下DisplayHelpVerbText方法的主体:
private void DisplayHelpVerbText(object options, Pair<MethodInfo, HelpVerbOptionAttribute> helpInfo, string verb)
{string helpText;if (verb == null){HelpVerbOptionAttribute.InvokeMethod(options, helpInfo, null, out helpText);}else{HelpVerbOptionAttribute.InvokeMethod(options, helpInfo, verb, out helpText);}if (_settings.HelpWriter != null){_settings.HelpWriter.Write(helpText);}
}
Since verb == null (see method call) we are interested in then-branch of the if statement. Although the situation is similar with the else branch, let's consider then-branch because in our particular case the execution went through it. Remember that helpInfo may be null.
由于动词== null (请参见方法调用),因此我们对if语句的then -branch感兴趣。 尽管形势与else分支类似, 那么,让我们来考虑分枝因为在我们的特定情况下执行通过它去。 请记住, helpInfo可以为null 。
Now let's look at the body of the HelpVerbOptionAttribute.InvokeMethod method. Actually, you have already seen it on the screenshot above:
现在让我们看一下HelpVerbOptionAttribute的主体。 InvokeMethod方法。 实际上,您已经在上面的屏幕截图中看到了它:
internal static void InvokeMethod(object target,Pair<MethodInfo, HelpVerbOptionAttribute> helpInfo,string verb,out string text)
{text = null;var method = helpInfo.Left;if (!CheckMethodSignature(method)){throw new MemberAccessException(SR.MemberAccessException_BadSignatureForHelpVerbOptionAttribute.FormatInvariant(method.Name));}text = (string)method.Invoke(target, new object[] { verb });
}
helpInfo.Left is called unconditionally, while helpInfo.Left是无条件调用的,而helpInfo may be helpInfo可以为null. The analyzer warned about it, and that's what happened.null 。 分析仪对此进行了警告,事实就是这样。
结论 (Conclusion)
It is nice that we managed to find an error in the source code of the library used in PVS-Studio with the help of PVS-Studio. I think this is a kind of the answer to the question «Does PVS-Studio find errors in PVS-Studio source code?». :) The analyzer can find errors not only in PVS-Studio code but also in the code of the used libraries.
很高兴我们借助PVS-Studio设法在PVS-Studio中使用的库的源代码中发现错误。 我认为这是对“ PVS-Studio是否在PVS-Studio源代码中发现错误?”这个问题的答案。 :)分析仪不仅可以在PVS-Studio代码中发现错误,而且可以在所用库的代码中发现错误。
Finally, I suggest you download the analyzer and try to check your project — what if you can find something interesting there too?
最后,我建议您下载分析器并尝试检查您的项目-如果在那里也可以找到有趣的东西怎么办?
翻译自: https://habr.com/en/company/pvs-studio/blog/462947/
关于PVS-Studio如何在用于... PVS-Studio的库中发现错误的故事相关推荐
- android so文件不混淆_Android studio 混淆打包时如何忽略依赖库中的第三方.so文件...
如题,今天在打包项目的时候各种报错,在debug的时候没有任何问题,那么很快定位到问题所在---第三方.so文件混淆出问题了.... 项目是在AS中编译的一个module,依赖了一个以前在Eclips ...
- Rekit Studio简介:用于React和Redux开发的真实IDE
by Nate Wang 内特·王 Rekit Studio简介:用于React和Redux开发的真实IDE (Introducing Rekit Studio: a real IDE for Rea ...
- 用于 Visual Studio Code 的 LaTeX Workshop
用于 Visual Studio Code 的 LaTeX Workshop 0. 简介 1. 特性 2. 安装和基本设置 2.1 需求 2.2 安装 2.3 设置 PATH 环境变量 2.4 使用 ...
- 【Android NDK 开发】Visual Studio 2019 使用 CMake 开发 JNI 动态库 ( 动态库编译配置 | JNI 头文件导入 | JNI 方法命名规范 )
文章目录 I . JNI 与 NDK 区别 II . Visual Studio 编译动态库 III. 配置 导入 jni.h 头文件 IV . IntelliJ IDEA Community Edi ...
- Android Studio(3)---Android Studio的配置
Android Studio配置 Android Studio 提供诸多向导和模板,可用于验证系统要求(例如 Java 开发工具包 (JDK) 和可用内存)和配置默认设置(例如优化的默认 Androi ...
- android studio创建构造方法,使用Android studio创建你的第一个项目
1.创建HelloWorld项目 任何编程语言写出的第一个程序毫无疑问都会是Hello World,这已经是自20世纪70年代一直流传下来的传统,在编程界已成为永恒的经典,那么今天就来使用Androi ...
- android studio官方教程 pdf,android studio教程pdf
android studio教程pdf [2021-02-13 02:22:01] 简介: php去除nbsp的方法:首先创建一个PHP代码示例文件:然后通过"preg_replace(& ...
- 【C++】Visual Studio教程(五) -安装 Visual Studio
00. 目录 文章目录 00. 目录 01. Visual Studio 2019 系统要求 02. Visual Studio安装 03. 预留 04. 预留 05. 附录 01. Visual S ...
- android 快速解析json数据搭配Gson库中Android Studio插件 GsonFormat
转:https://www.jianshu.com/p/d34c5e7c8227 更简洁的文章:http://www.cnblogs.com/foxy/p/7825380.html 如果对json解析 ...
最新文章
- 中国交通标志识别,德国交通标志识别
- 用git发patch
- java创建对象new后面为啥可以传入参数_来复习一下Java的对象知识
- EDAS提交论文字体未嵌入
- Linux软中断、tasklet和工作队列
- mysql5.6找不到int_为什么在mysql 5.6中,在int字段上自动递增跳过“2147483646”?
- ktv服务器操作系统,开源ktv客户端服务器系统
- 最常用的五种数据分析方法,建议收藏!
- cad卸载_IT运维:CAD卸载不彻底,无法重装?
- 幸运彩票 分数 15作者 陈越单位 浙江大学
- 可靠性(reliability)弱点度量
- 阿里云新优惠活动,幸运券免费领取
- uni app video、视频播放开发
- android接入支付宝自动续费,APP是如何实现自动续费的?
- blender合并物体后材质丢失问题的解决办法
- 骞云科技DevOps实践
- 区块链和公益怎么如何融合到一起
- 编译安装Gearman
- Shader Graph 小草顶点动画
- Anaconda环境下离线安装dlib库,在线安装很多问题报错,离线安装速度快稳定
热门文章
- jquery给一个li标签添加和去掉class属性
- uni-app 唤醒调用第三方app并传参(一 显示调用)
- docker 部署 mkdocs (mkdocs-material)
- Android合成Gif
- java objectpool_java objectpool 对象池
- 前端图片拖拽,滚轮放大缩小
- 中国AI+营销市场研究报告
- 独家报道:CentOS7[修改主机名以及IP对应关系]
- wise care 365下载|wise care 365 pro绿色便携版v5.0.1下载
- Ubuntu18.04安装basler相机的ROS驱动程序pylon_ros_camera