IList对象排序算法
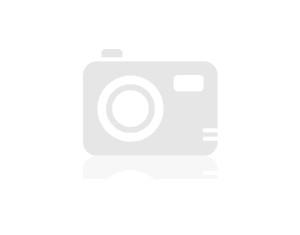
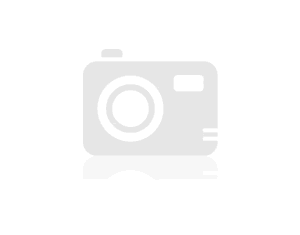
/**********************************************************************************
* ClassName: IListSort
* Description: IList排序类
* MemberVariable:
* Functions: 暂时使用插入排序方法
* Modify information:
* Date ModifyFlag Charged by Liujianming
* 2008年11月18日17:39:30 New Liujianming Create Class
*
* ********************************************************************************/
using System;
using System.Collections.Generic;
using System.Text;
using System.Reflection;
namespace Sobey.MAM.WebPortal
{
///
/// IList排序类
///
///
public class IListSort
{
private string _propertyName;
private bool _sortBy = true;
private IList _list;
///
/// 构造函数
///
/// 排序的Ilist
/// 排序字段属性名
/// true升序 false 降序 不指定则为true
public IListSort(IList list, string propertyName, bool sortBy)
{
_list = list;
_propertyName = propertyName;
_sortBy = sortBy;
}
///
/// 构造函数
///
/// 排序的Ilist
/// 排序字段属性名
/// true升序 false 降序 不指定则为true
public IListSort(IList list, string propertyName)
{
_list = list;
_propertyName = propertyName;
_sortBy = true;
}
///
/// IList
///
public IList List
{
get { return _list; }
set { _list = value; }
}
///
/// 排序字段属性名
///
public string PropertyName
{
get { return _propertyName; }
set { _propertyName = value; }
}
///
/// true升序 false 降序
///
public bool SortBy
{
get { return _sortBy; }
set { _sortBy = value;}
}
///
/// 排序,插入排序方法
///
///
public IList Sort()
{
if (_list.Count == 0) return _list;
for (int i = 1; i < _list.Count; i++) {
T t = _list[i];
int j = i;
while ((j > 0) && Compare(_list[j - 1], t) < 0) {
_list[j] = _list[j - 1];
--j;
}
_list[j] = t;
}
return _list;
}
///
/// 比较大小 返回值 小于零则X小于Y,等于零则X等于Y,大于零则X大于Y
///
///
///
///
private int Compare(T x, T y)
{
if (string.IsNullOrEmpty(_propertyName)) throw new ArgumentNullException("没有指字对象的排序字段属性名!");
PropertyInfo property = typeof( T ).GetProperty(_propertyName);
if (property == null) throw new ArgumentNullException("在对象中没有找到指定属性!");
switch (property.PropertyType.ToString()) {
case "System.Int32" :
int int1 = 0;
int int2 = 0;
if (property.GetValue(x, null) != null) {
int1 = Convert.ToInt32(property.GetValue(x, null));
}
if (property.GetValue(y, null) != null) {
int2 = Convert.ToInt32(property.GetValue(y, null));
}
if (_sortBy) {
return int2.CompareTo(int1);
}
else {
return int1.CompareTo(int2);
}
break;
case "System.Double":
double double1 = 0;
double double2 = 0;
if (property.GetValue(x, null) != null) {
double1 = Convert.ToDouble(property.GetValue(x, null));
}
if (property.GetValue(y, null) != null) {
double2 = Convert.ToDouble(property.GetValue(y, null));
}
if (_sortBy) {
return double2.CompareTo(double1);
}
else {
return double1.CompareTo(double2);
}
break;
case "System.String" :
string string1 = string.Empty;
string string2 = string.Empty;
if (property.GetValue(x, null) != null) {
string1 = property.GetValue(x, null).ToString();
}
if (property.GetValue(y, null) != null) {
string2 = property.GetValue(y, null).ToString();
}
if (_sortBy) {
return string2.CompareTo(string1);
}
else {
return string1.CompareTo(string2);
}
break;
case "System.DateTime":
DateTime DateTime1 = DateTime.Now;
DateTime DateTime2 = DateTime.Now;
if (property.GetValue(x, null) != null) {
DateTime1 = Convert.ToDateTime(property.GetValue(x, null));
}
if (property.GetValue(y, null) != null) {
DateTime2 = Convert.ToDateTime(property.GetValue(y, null));
}
if (_sortBy) {
return DateTime2.CompareTo(DateTime1);
}
else {
return DateTime1.CompareTo(DateTime2);
}
break;
}
return 0;
}
}
}
转载于:https://www.cnblogs.com/yupipi520/archive/2008/12/31/1365727.html
IList对象排序算法相关推荐
- java冒泡遍历对象_Java经典排序算法(冒泡、选择、插入)
排序算法说明 排序说明 对一序列对象根据某个关键字进行排序. 术语说明 稳定:如果a原本在b前面,而a=b,排序之后a仍然在b的前面: 不稳定:如果a原本在b的前面,而a=b,排序之后a可能会出现在b ...
- java python算法_用Python,Java和C ++示例解释的排序算法
java python算法 什么是排序算法? (What is a Sorting Algorithm?) Sorting algorithms are a set of instructions t ...
- 在遗传算法中出现等式约束_排序算法中的稳定性-等式的处理
在遗传算法中出现等式约束 by Onel Harrison 通过Onel Harrison 排序算法中的稳定性-等式的处理 (Stability in Sorting Algorithms - A T ...
- 漫画 | 程序媛小姐姐带你一次了解什么是排序算法
来源 | 小齐本齐 封图 | CSDN 付费下载自视觉中国 插入排序 借用<算法导论>里的例子,就是我们打牌的时候,每新拿一张牌都会把它按顺序插入,这,其实就是插入排序. 齐姐声明:虽然我 ...
- 3分钟快速实现:9种经典排序算法的可视化
作者 | 爱笑的眼睛 来源 | 恋习Python(ID:sldata2017) 最近在某网站上看到一个视频,是关于排序算法的可视化的,看着挺有意思的,也特别喜感. ▼ 6分钟演示15种排序算法 不知道 ...
- scala编写排序算法
1.产生随机序 def RandomList(n: Int) = Seq.fill(n)(scala.util.Random.nextInt(n))def RandomDiffList(n: Int) ...
- 八大排序算法合集 (归并排序、交换排序、插入排序、选择排序......)
目录 一.归并排序 二.交换排序 1.快速排序 2.冒泡排序 三.插入排序 1.直接插入排序(基于顺序查找) 2.折半插入排序(基于折半查找) 3.希尔排序(基于逐趟缩小增量) 四.选择排序 0.直接 ...
- 函数对象、 函数对象与容器、函数对象与算法
一.函数对象 1.函数对象(function object)也称为仿函数(functor) 2.一个行为类似函数的对象,它可以没有参数,也可以带有若干参数. 3.任何重载了调用运算符operator( ...
- 3min利用Python实现9种经典排序算法可视化!(附源代码)
来源:恋习Python 本文附视频,建议收藏. 本文为你分享实现9种经典排序算法可视化的方法,3分钟即可实现. [导 读]近在某网站上看到一个视频,是关于排序算法的可视化的,看着挺有意思的,也特别喜感 ...
最新文章
- 2015/5/9站立会议(补发)
- 国防科大计算机专业分数线,2018国防科技大学各省录取分数线_2017年国防科大录取线...
- mysql 140824,Oracle 12c创建可插拔数据库(PDB)及用户
- scrollLeft,scrollWidth,clientWidth,offsetWidth之完全详解
- HTML5中的本地数据库-Web SQL Database
- 吴恩达神经网络1-2-2_图神经网络进行药物发现-第1部分
- python表格写操作单元格合并
- 【华为云技术分享】玩转华为物联网IoTDA服务系列三-自动售货机销售分析场景示例
- 爱企人事工资管理系统 v8.1 免费下载
- Spark核心概念与案例拆解
- 各省份国内、入境旅游人数 (2007-2018年)
- Creo:Creo2.0安装实例教程之图文详细攻略
- paddlepaddle波士顿房价预测
- github-上传本地代码到github仓库
- Xshell如何设置快捷复制、粘贴热键
- 一个过不了情关的男人!!
- 怎样进行https证书检查
- 日历农历vue包括24节气等内容
- HEVC新技术(一):基于MVC的AMVP技术
- rem与px之间的转换
热门文章
- 巡查准确率怎么算_【达睿原创】需求预测准确率,你怎么看 ?
- rfid在高速公路管理中的应用_RFID亮灯电子标签在仓储管理中的应用
- java学习之------位运算符实现两值交换
- PAID Network宣布已获币安DeFi加速器基金投资
- Amber Group与1Token达成合作,引入CAM系统加码机构级财务方案
- Cover开启投票是否对Yearn漏洞提供保险
- 慢雾安全工程师:安全审计是目前保护 DeFi 项目安全最高性价比的方式
- SAP License:SAP顾问行业的生活状态实录,新人值得一看!
- 在线教育、直播教育、课程直播、订单系统、老师介绍、收入提现、在线学习、业绩统计、课程统计、选老师、选课程、作业管理、课程管理、报名统计、在线教育管理系统、axure原型、rp源文件
- Linux中,Tomcat 怎么承载高并发(深入Tcp参数 backlog)