NET|C#生成PDF文件
转自:
项目需要在线生成PDF文件,我首先考虑采用itextsharp控件来实现。具体方法参考 https://sourceforge.net/projects/itextsharp/
1.首先利用nuget 引入该控件包。
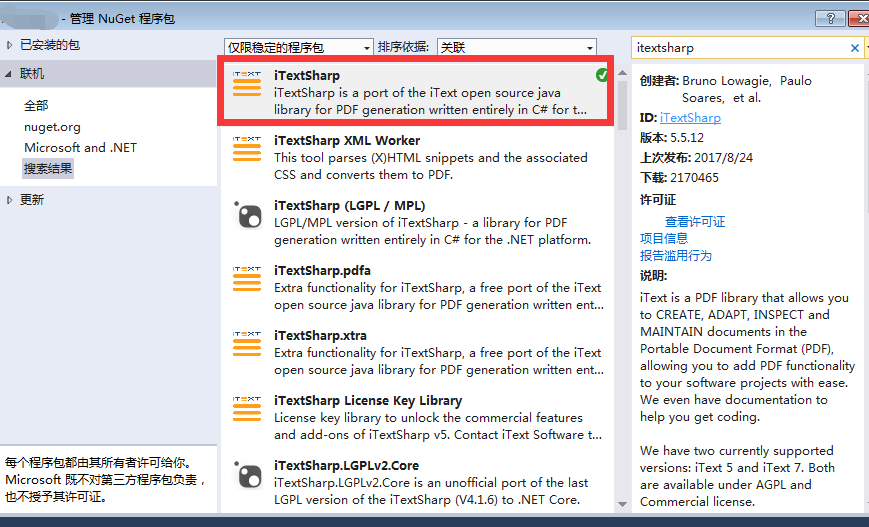
2、然后直接创建就可以了。
using iTextSharp.text;
using iTextSharp.text.pdf;
using System;
using System.Collections.Generic;
using System.Data;
using System.IO;
using System.Linq;
using System.Text;
using System.Web;public class CreatePDF
{private static CreatePDF instance;public static CreatePDF GetInstance(){if (instance == null){instance = new CreatePDF();}return instance;}private static Document doc;//string fontdb = AppDomain.CurrentDomain.BaseDirectory + "Template\\msyh.ttc";//private static BaseFont bf = BaseFont.CreateFont(@"C://Windows/Fonts/simsun.ttc,0", BaseFont.IDENTITY_H, BaseFont.EMBEDDED);static string fontdb = (AppDomain.CurrentDomain.BaseDirectory + "Template\\msyh.ttf").Replace("\\", "/");private static BaseFont bf = BaseFont.CreateFont(fontdb + "", BaseFont.IDENTITY_H, BaseFont.EMBEDDED);//四种字体private static Font fontBig = new Font(bf, 14, Font.BOLD);private static Font fontSmall = new Font(bf, (float)10.5, Font.BOLD);private static Font fontSmallNoBold = new Font(bf, (float)10.5);private static float IndentationLeft = 50;//距左边距//如果要传参数进来,可自定义public string GeneratePDF(string name){doc = new Document(PageSize.A4);string filePath = string.Empty;try{//MemoryStream ms2 = new MemoryStream();string fileName = string.Format("{0}.pdf", DateTime.Now.ToString("yyyyMMddHHmmss"));filePath = AppDomain.CurrentDomain.BaseDirectory + "Template\\" + fileName;FileStream fs = new FileStream(filePath, FileMode.Create);//创建临时文件,到时生成好后删除PdfWriter writer = PdfWriter.GetInstance(doc, fs);writer.CloseStream = false;//把doc内容写入流中doc.Open();//核心操作CreateLine(name);//生成一条下横线#region 添加水印string waterMarkName = "机密";#endregiondoc.Close();MemoryStream ms = new MemoryStream();if (fs != null){byte[] bytes = new byte[fs.Length];//定义一个长度为fs长度的字节数组fs.Read(bytes, 0, (int)fs.Length);//把fs的内容读到字节数组中ms.Write(bytes, 0, bytes.Length);//把字节内容读到流中fs.Flush();fs.Close();}MemoryStream waterMS = SetWaterMark(ms, filePath, waterMarkName);//先生成水印,再删除临时文件//if (File.Exists(filePath))//判断临时文件是否存在,如果存在则删除//{// File.Delete(filePath);// GC.Collect();//回收垃圾//}//SendFile(fileName, waterMS);//把PDF文件发送回浏览器}catch (DocumentException ex){throw new Exception(ex.Message);}return filePath;}#region 生成一条横线private static void CreateLine(string name){PdfPTable table = new PdfPTable(1);//一个单元格的PdfPCell cell = new PdfPCell();Image gif = Image.GetInstance(AppDomain.CurrentDomain.BaseDirectory + "Template\\logo.png");gif.ScaleAbsoluteWidth(100);cell.AddElement(gif);cell.BorderWidth = 0f;cell.BorderWidthBottom = 0.2f;table.AddCell(cell);doc.Add(table);}#endregion#region 生成页码private static void AddPageNumberContent(){var content = new Paragraph("共 页 第 页", fontSmall);content.IndentationRight = IndentationLeft + 20;content.Alignment = 2; //居左doc.Add(content);}#endregion#region 生成单元格private static PdfPCell GetPdfCell(string content, Font font, int horizontalAlignment){Paragraph paragraph = new Paragraph(content, font);paragraph.FirstLineIndent = 2;paragraph.SetLeading(10, 20);paragraph.SpacingAfter = 10;PdfPCell cell = new PdfPCell(paragraph);cell.HorizontalAlignment = horizontalAlignment;//水平位置cell.VerticalAlignment = Element.ALIGN_CENTER;//垂直居中cell.MinimumHeight = 20;//单元格的最小高度cell.Border = 0;return cell;}#endregion#region 生成水印private static MemoryStream SetWaterMark(MemoryStream ms, string filePath, string waterMarkName, string waterMarkAddr = null){MemoryStream msWater = new MemoryStream();PdfReader pdfReader = null;PdfStamper pdfStamper = null;try{pdfReader = new PdfReader(filePath);pdfStamper = new PdfStamper(pdfReader, msWater);int total = pdfReader.NumberOfPages + 1;//获取PDF的总页数iTextSharp.text.Rectangle psize = pdfReader.GetPageSize(1);//获取第一页float width = psize.Width;//PDF页面的宽度,用于计算水印倾斜float height = psize.Height;PdfContentByte waterContent;BaseFont basefont = BaseFont.CreateFont(@"C:\WINDOWS\Fonts\SIMFANG.TTF", BaseFont.IDENTITY_H, BaseFont.EMBEDDED);PdfGState gs = new PdfGState();for (int i = 1; i < total; i++){waterContent = pdfStamper.GetOverContent(i);//在内容上方加水印//透明度waterContent.SetGState(gs);//开始写入文本waterContent.BeginText();waterContent.SetColorFill(BaseColor.RED);waterContent.SetFontAndSize(basefont, 18);waterContent.SetTextMatrix(0, 0);if (waterMarkAddr == null || waterMarkAddr == ""){waterContent.ShowTextAligned(Element.ALIGN_CENTER, waterMarkName, width / 2, height / 2, 55);}else{waterContent.ShowTextAligned(Element.ALIGN_CENTER, waterMarkName, width / 2, height / 2 + 100, 55);waterContent.ShowTextAligned(Element.ALIGN_CENTER, waterMarkAddr, width / 2, height / 2 - 100, 55);}waterContent.EndText();}}catch (Exception){return ms;}finally{if (pdfStamper != null)pdfStamper.Close();if (pdfReader != null)pdfReader.Close();}return msWater;}#endregion//-----------------------发送PDF文件回浏览器端----------------------public static void SendFile(string fileName, MemoryStream ms, Encoding encoding = null){fileName = (fileName + "").Replace(" ", "");encoding = encoding ?? Encoding.UTF8;if (ms != null && !string.IsNullOrEmpty(fileName)){System.Web.HttpResponse response = System.Web.HttpContext.Current.Response;response.Clear();response.Charset = encoding.BodyName;// "utf-8";if (!HttpContext.Current.Request.UserAgent.Contains("Firefox") && !HttpContext.Current.Request.UserAgent.Contains("Chrome")){fileName = HttpUtility.UrlEncode(fileName, encoding);}response.AddHeader("Content-Disposition", "attachment;filename=" + fileName);//为了解决打开,导出NPOI生成的xlsx文件时,提示发现不可读取内容。if (!(fileName + "").ToLower().EndsWith(".xlsx")){response.AddHeader("Content-Type", "application/octet-stream");response.BinaryWrite(ms.GetBuffer());}else{response.BinaryWrite(ms.ToArray());}ms.Close();ms = null;response.Flush();response.End();}}
}
特别需要注意一点的是,如果对字体有特殊要求,可以将字库文件放在项目目录下,但字库的文件类型不同,引用时略有差别。
ttc文件
BaseFont.CreateFont(@"C://Windows/Fonts/simsun.ttc,0", BaseFont.IDENTITY_H, BaseFont.EMBEDDED);
ttf文件
BaseFont.CreateFont(@"C://Windows/Fonts/simsun.ttf", BaseFont.IDENTITY_H, BaseFont.EMBEDDED);
这种模式的弊端在于调整文档的格式太痛苦了,你想按照预定的模板生成pdf,格式要按照他的机制一点点写,如果是篇幅比较长的文档,耐心和时间缺一不可。
在我埋头写了一天之后,同事路过看到我一脸狰狞,细问之,马上丢给我一个包,十分钟后搞定。没有广告,工具类马上贴上来。
本方法依赖于Aspose.Words,因为版权问题,不要直接用nuget引入,需要去网上down一个破解版。然后将需要生成的文件内容置于一个word中,相应参数用字符标识后,方便替换。最后加入以下工具类直接调用就OK了。
(破解dll可以在这里自取 链接: https://pan.baidu.com/s/1o7XpCqI 密码: kwpt)
CrackWord.cs
using System;
using System.Reflection;class CrackWord
{/// <summary>/// 16.10.1.0/// </summary>public static void Crack()//使用前调用一次即可{string[] stModule = new string[8]{"\u000E\u2008\u200A\u2001","\u000F\u2008\u200A\u2001","\u0002\u200A\u200A\u2001","\u000F","\u0006","\u000E","\u0003","\u0002"};Assembly assembly = Assembly.GetAssembly(typeof(Aspose.Words.License));Type typeLic = null, typeIsTrial = null, typeHelper = null;foreach (Type type in assembly.GetTypes()){if ((typeLic == null) && (type.Name == stModule[0])){typeLic = type;}else if ((typeIsTrial == null) && (type.Name == stModule[1])){typeIsTrial = type;}else if ((typeHelper == null) && (type.Name == stModule[2])){typeHelper = type;}}if (typeLic == null || typeIsTrial == null || typeHelper == null){throw new Exception();}object lic = Activator.CreateInstance(typeLic);int findCount = 0;foreach (FieldInfo field in typeLic.GetFields(BindingFlags.NonPublic | BindingFlags.Static | BindingFlags.Instance)){if (field.FieldType == typeLic && field.Name == stModule[3]){field.SetValue(null, lic);++findCount;}else if (field.FieldType == typeof(DateTime) && field.Name == stModule[4]){field.SetValue(lic, DateTime.MaxValue);++findCount;}else if (field.FieldType == typeIsTrial && field.Name == stModule[5]){field.SetValue(lic, 1);++findCount;}}foreach (FieldInfo field in typeHelper.GetFields(BindingFlags.NonPublic | BindingFlags.Static | BindingFlags.Instance)){if (field.FieldType == typeof(bool) && field.Name == stModule[6]){field.SetValue(null, false);++findCount;}if (field.FieldType == typeof(int) && field.Name == stModule[7]){field.SetValue(null, 128);++findCount;}}if (findCount < 5){throw new NotSupportedException("无效的版本");}}
}
ReplaceAndInsertImage.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Aspose.Words;
using Aspose.Words.Replacing;public class ReplaceAndInsertImage : IReplacingCallback
{/// <summary>/// 需要插入的图片路径/// </summary>public string Url { get; set; }public ReplaceAndInsertImage(string url){this.Url = url;}public ReplaceAction Replacing(ReplacingArgs e){//获取当前节点var node = e.MatchNode;//获取当前文档Document doc = node.Document as Document;DocumentBuilder builder = new DocumentBuilder(doc);//将光标移动到指定节点builder.MoveTo(node);//插入图片builder.InsertImage(Url);return ReplaceAction.Replace;}
}
Cv.cs 调用生成类
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Text.RegularExpressions;
using System.Threading.Tasks;
using Aspose.Words;public class Cv
{/// <summary>/// 生成录用offer文档/// </summary>/// <param name="source">模板文件</param>/// <param name="filespath">生成的PDF目录</param>/// <returns></returns>public static void Run(string source, string filespath,){var dic = new Dictionary<string, string>();//替换模板文件的参数dic.Add("{xingming}","参数");CrackWord.Crack();Aspose.Words.Document doc = new Aspose.Words.Document(source);foreach (var key in dic.Keys){
#pragma warning disable 618doc.Range.Replace(key, dic[key], false, false);
#pragma warning restore 618}doc.Save(filespath, SaveFormat.Pdf);}
}
source 为模板文件目录,本例中为word 文件。
NET|C#生成PDF文件相关推荐
- python使用fpdf生成pdf文件章节(chapter),包含:页眉、页脚、章节主题、数据排版等;
python使用fpdf生成pdf文件章节(chapter),包含:页眉.页脚.章节主题.数据排版等: #仿真数据 The year 1866 was marked by a bizarre deve ...
- python使用fpdf生成pdf文件:配置多种语言字体写入多种文字
python使用fpdf生成pdf文件:配置多种语言字体写入多种文字 目录
- 一步快速将Smartform output转成生成PDF文件
世间竟有如此盖世神功!最简单的smart form output转化成 PDF文件的方式. Form打印预览界面在事务栏输入T-code:PDF!,注意!为半角英文状态下的感叹号. 输入T-cod ...
- 安装texlive并用latex编写一段中文,最后生成pdf文件
安装texlive并用latex编写一段中文,最后生成pdf文件 **#一.下载安装(**链接https://tug.org/texlive/) ##1.第一步 ##2.第二步 ##3.第三步 ##4 ...
- 小容量单片机生成pdf文件
工作上要求使用小容量单片机生成直接生成pdf文件. 经过一段时间的摸索,其中参考了libharu,库太大,不适合在单片机上使用 页参考了与非网上一位前辈的库,占用的RAM太大,不适合小容量单片机, 主 ...
- php输出PDF的文件流_怎么用PHP在HTML中生成PDF文件
译文:使用PHP在html中生成PDF 译者:dwqs 利用PHP编码生成PDF文件是一个非常耗时的工作.在早期,开发者使用PHP并借助FPDF来生成PDF文件.但是如今,已经有很多函数库可以使用了, ...
- python数据生成pdf,Python生成pdf文件的方法
摘要:这篇Python开发技术栏目下的"Python生成pdf文件的方法",介绍的技术点是"python生成pdf文件.python生成pdf.生成pdf文件.Pytho ...
- java调用wkhtmltopdf生成pdf文件,美观,省事
最近项目需要导出企业风险报告,文件格式为pdf,于是搜了一大批文章都是什么Jasper Report,iText ,flying sauser ,都尝试了一遍,感觉不是我想要的效果, 需要自己调整好多 ...
- django生成文件txt、pdf(在生成 PDF 文件之前,需要安装 ReportLab 库)
from django.http import HttpResponse def download_file(request): # Text file #response = HttpRespons ...
- itextpdf添加表格元素_itext生成pdf文件-表格
生成pdf常用的插件有iReport.和itext,这里将使用itext生成pdf文件. 多于的话不说直接上demo和需要的jar,如果pdf中有图片要画的话可以用jfreeChart画. packa ...
最新文章
- ios水果风暴游戏源码项目下载
- 架构师之路 — 数据库设计 — 关系型数据库应用程序设计
- 2.5亿个整数中找出不重复的整数
- java的query_Java-Query
- CTF C#逆向Reverse
- 万达电影携手神策数据 数据赋能打造全球领先电影生活生态圈
- IT兄弟连 Java语法教程 Java的发展历程
- [HEOI2013] SAO(dp + 组合数 + 前缀和)
- 什么函数是回调函数?
- java 替换所有中文_java 替换中文
- JAVA数据库宾馆住宿系统_java+数据库 宾馆客房管理系统
- 说说我出道后的处女作:剪贴板神器 iPaste
- 6、Latex学习笔记之参考文献篇
- 如何做一名优秀的工程师
- 分享11款Steam推理游戏
- 京东获取商品历史价格信息 API接口
- cocos2dx.3.17中用VS2017启动本地windows调试器出现脚本错误解决办法
- linux下XAMP集成开发环境搭建流程总结
- 企业邮箱密码怎么找回?
- Java 访问 HDFS操作