Spring 4 + Reactor Integration Example--转
原文地址:http://www.concretepage.com/spring-4/spring-4-reactor-integration-example
Reactor is a framework to make event driven programming much easier. This is based on Reactor Design Pattern. Reactor is good for asynchronous applications on the JVM. Here we will create asynchronous and event driven application using Spring 4 and Reactor. Reactor uses Selectors, Consumers and Events as core module. Consumer is event consumer which needs to be notified for the event. Reactor is event gateway where event consumers are registered with a notification key. Selector is an abstraction to find consumer by invoking event. Find the example for detailed understanding.
Software Required to Run Example
To run the example we need the following software.
1. JDK 6
2. Gradle
3. Eclipse
Project Structure in Eclipse
Find our demo project structure in eclipse.
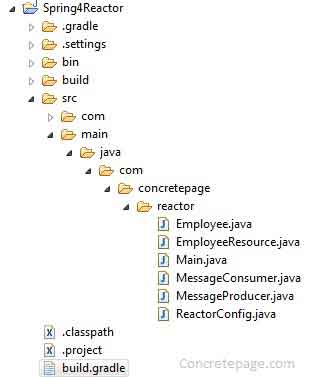
Java Class for JSON
For the event driven example, we have created sample page that will return the JSON as below.
{"result":"success","employee":{"id":1,"name":"Ram"}}
To get this output we are using local URL as http://localhost:8080/empdata.jsp
To consume the JSON, we have two classes as below.
Employee.java
package com.concretepage.reactor; import com.fasterxml.jackson.annotation.JsonIgnoreProperties; @JsonIgnoreProperties(ignoreUnknown=true) public class Employee { private Integer id; private String name; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
Find the EmployeeResource.java.
EmployeeResource.java
package com.concretepage.reactor; public class EmployeeResource { private String result; private Employee employee; public String getResult() { return result; } public void setResult(String result) { this.result = result; } public Employee getEmployee() { return employee; } public void setEmployee(Employee employee) { this.employee = employee; } }
Create Consumer using reactor.function.Consumer
To create the consumer, our class must implement reactor.function.Consumer. We need to override accept method which has the argument as reactor.event.Event. To get the event data we can use the method as Event.getData. Here in this method, we are accessing a REST URL that will return the JSON data.
MessageConsumer.java
package com.concretepage.reactor; import java.util.concurrent.CountDownLatch; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.client.RestTemplate; import reactor.event.Event; import reactor.function.Consumer; public class MessageConsumer implements Consumer<Event<Integer>> { @Autowired CountDownLatch latch; RestTemplate restTemplate = new RestTemplate(); @Override public void accept(Event<Integer> event) { EmployeeResource empResource = restTemplate.getForObject("http://localhost:8080/empdata.jsp", EmployeeResource.class); System.out.println("Employee " + event.getData() + ":" + empResource.getEmployee().getName()); latch.countDown(); } }
Create Producer
In the producer class, we are notifying consumer with a key and an event that is ready to be processed.
MessageProducer.java
package com.concretepage.reactor; import java.util.concurrent.CountDownLatch; import java.util.concurrent.atomic.AtomicInteger; import org.springframework.beans.factory.annotation.Autowired; import reactor.core.Reactor; import reactor.event.Event; public class MessageProducer { @Autowired Reactor reactor; @Autowired CountDownLatch latch; public void publishEmployee(int numberOfEmp) throws InterruptedException { AtomicInteger counter = new AtomicInteger(1); for (int i=0; i < numberOfEmp; i++) { reactor.notify("employees", Event.wrap(counter.getAndIncrement())); } latch.await(); System.out.println("-------Done-------"); } }
Configuration Class for Reactor
We need to create a reactor.core.Reactor bean that needs reactor.core.Environment as an argument.
ReactorConfig.java
package com.concretepage.reactor; import java.util.concurrent.CountDownLatch; import org.springframework.boot.autoconfigure.EnableAutoConfiguration; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import reactor.core.Environment; import reactor.core.Reactor; import reactor.core.spec.Reactors; @Configuration @EnableAutoConfiguration public class ReactorConfig { private static final int NUMBER_OF_EMP = 5; @Bean Environment env() { return new Environment(); } @Bean Reactor reactor(Environment env) { return Reactors.reactor() .env(env) .dispatcher(Environment.THREAD_POOL) .get(); } @Bean MessageConsumer consumer(){ return new MessageConsumer(); } @Bean MessageProducer producer(){ return new MessageProducer(); } @Bean public CountDownLatch latch() { return new CountDownLatch(NUMBER_OF_EMP); } }
Main Class to Run Example
Find the main class to run the example. Reactor provides Selectors to run our asynchronous and event-driven application. Here we using $ selector. Reactor.on method registers a consumer that is triggered when notification matches the given selector.
Main.java
package com.concretepage.reactor; import static reactor.event.selector.Selectors.$; import org.springframework.context.annotation.AnnotationConfigApplicationContext; import reactor.core.Reactor; public class Main { public static void main(String[] args) throws InterruptedException { final int NUMBER_OF_EMP = 5; AnnotationConfigApplicationContext ctx = new AnnotationConfigApplicationContext(); ctx.register(ReactorConfig.class); ctx.refresh(); Reactor reactor = (Reactor)ctx.getBean("reactor"); MessageConsumer consumer = (MessageConsumer)ctx.getBean("consumer"); MessageProducer publisher = (MessageProducer)ctx.getBean("producer"); reactor.on($("employees"), consumer); publisher.publishEmployee(NUMBER_OF_EMP); } }
Find the output.
Employee 2:Ram Employee 4:Ram Employee 3:Ram Employee 5:Ram Employee 1:Ram -------Done-------
Gradle for Spring and Reactor JAR Depedency
Find the gradle file to resolve the JAR dependency.
build.gradle
apply plugin: 'java' apply plugin: 'eclipse' archivesBaseName = 'Concretepage' version = '1.0-SNAPSHOT' repositories { maven { url "https://repo.spring.io/libs-release" } mavenLocal() mavenCentral() } dependencies { compile 'org.springframework.boot:spring-boot-starter:1.2.0.RELEASE' compile 'org.projectreactor.spring:reactor-spring-context:1.1.3.RELEASE' compile 'org.springframework:spring-web:4.1.3.RELEASE' compile 'com.fasterxml.jackson.core:jackson-databind:2.5.0-rc1' compile 'org.springframework.data:spring-data-commons:1.9.1.RELEASE' compile 'org.springframework.boot:spring-boot-starter-security:1.2.0.RELEASE' }
Now we are done. Enjoy Learning.
转载于:https://www.cnblogs.com/davidwang456/p/5690525.html
Spring 4 + Reactor Integration Example--转相关推荐
- SpringOne 2017:与Pivotal聊大会、Spring、Reactor、WebFlux及其他
在旧金山举行的SpringOne平台大会上,我们采访了来自Pivotal的Pieter Humphrey和Simon Basle. \\ InfoQ:欢迎二位.你们能介绍一下你们是做什么的吗? \\ ...
- Spring,Reactor和ElasticSearch:从回调到React流
Spring 5(以及Boot 2,将在数周内到货)是一次革命. 不是" XML上的注释 "或" Java上的注释类 "的革命. 这是一个真正的革命性框架,可以 ...
- Spring,Reactor和ElasticSearch:使用伪造的测试数据进行标记
在上一篇文章中,我们创建了一个从ElasticSearch的API到Reactor的Mono的简单适配器,如下所示: import reactor.core.publisher.Mono;privat ...
- Spring,Reactor和ElasticSearch:从回调到反应流
Spring 5(以及Boot 2,在数周之内到货)是一次革命. 不是" XML上的注释 "或" Java上的注释类 "的革命. 这是一个真正的革命性框架,可以 ...
- Spring Security(三十六):12. Spring MVC Test Integration
Spring Security provides comprehensive integration with Spring MVC Test Spring Security提供与Spring MVC ...
- 实战 Spring Cloud Gateway 之限流篇
来源:https://www.aneasystone.com/archives/2020/08/spring-cloud-gateway-current-limiting.html 话说在 Sprin ...
- spring框架_Spring框架
spring框架 Spring Framework is one of the most popular Java EE frameworks. Spring框架是最受欢迎的Java EE框架之一. ...
- Spring Cloud Sleuth 配置说明
名称 默认值 说明 spring.sleuth.async.configurer.enabled true 启用默认的AsyncConfigurer. spring.sleuth.async.enab ...
- 这可能是全网Spring Cloud Gateway限流最完整的方案了!
作者:aneasystone https://www.aneasystone.com/ 话说在 Spring Cloud Gateway 问世之前,Spring Cloud 的微服务世 ...
最新文章
- [BJDCTF2020]EzPHP 1
- UVA-806 Spatial Structures (四分树)
- 【小白学习C++ 教程】二十、C++ 中的auto关键字
- GCDWebUploader支持iOS进入后台后仍然可以进行传输
- SAP ABAP实用技巧介绍系列之 使用simple transformation的mapping功能
- android自定义view生命周期,android基础之自定义view
- Idea运行项目报错:java.lang.OutOfMemoryError: Java heap space/ java.lang.OutOfMemoryError: GC overhead 解决方法
- idea卸载不干净怎么办_家里拖地老是不干净怎么办,来跟我学!
- 关于spark写入文件至文件系统并制定文件名之自定义outputFormat
- Jupyter Notebook——如何快速地以当前文件夹目录打开 Jupyter Notebook
- Spring MVC + freemarker实现半自动静态化
- 牛顿法、梯度下降法原理及Python编程应用
- linux kprobe rootkit学习
- django models 数据库 update_or_create 更新或者插入
- 集成mybatis-generator-maven-plugin报错A required class was missing while ..org/mybatis/generator/api/dom
- Exploiting Spatial Structure for Localizing Manipulated Image Regions
- 关于go module
- 动态海报,一次 Computational Design 实验
- ThinkPHP5.1.x 框架源码分析之框架的灵魂
- could not resolve dependency: npm err! peer react@“^16.8.0 || ^17.0.0“ from @material-ui/core@4.12.4
热门文章
- python调用命令行获取pid_python 使用标准库根据进程名获取进程的pid
- php 子类名,php的继承方法获取子类名
- 51单片机的中断系统
- SQLServer中的数据类型
- C++中多态的概念和意义
- java script isblank_java判断一个字符串是否为空,isEmpty和isBlank的区别
- gitlab更新配置无效_GitMaster 发布 v1.11.0 版本,支持 GitLab 多级分组,Gist支持文件列表...
- 计算机视觉编程——增强现实基础
- qq分享 设备未授权报错解决方案_金融行业思科设备典型网络故障案例:76系列典型案例(四)...
- NTU 笔记 6422quiz 复习(1~3节)