中微子超光速_定制中微子皮棉
中微子超光速
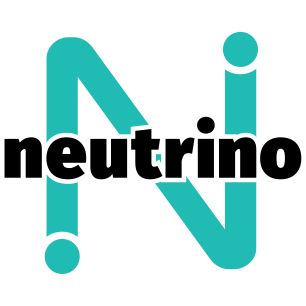
Last week my friend Eli Perelman shared Modern JavaScript Apps with Neutrino, an awesome new Node.js tool for creating amazing apps with minimal fuss. No need to learn webpack, scour babel plugins, or search for what exactly is required to get a React.js app up and running -- just install Neutrino and go! I've been super impressed with Eli's work and the ease of development for customization.
上周,我的朋友Eli Perelman 与Neutrino共享了Modern JavaScript Apps,Neutrono是一个很棒的新Node.js工具,用于以最小的麻烦创建出色的应用程序。 无需学习webpack,搜索babel插件或搜索启动和运行React.js应用程序所需的确切内容-只需安装Neutrino即可! Eli的工作和定制开发的简易性给我留下了深刻的印象。
One important customization for me was the ability to modify default linting rules and running the lint routine from command line when I wanted to. Neutrino does provide a default ESLint rule set, and does lint while you modify your code, but testing if linting passes within CI is also important. Let's look at how we can create custom linting rules with our own custom preset!
对我来说,一项重要的自定义功能是能够修改默认的掉毛规则并在需要时从命令行运行lint例程。 Neutrino确实提供了默认的ESLint规则集,并且在您修改代码时也提供了lint,但是测试是否在CI中通过掉毛也很重要。 让我们看看如何使用我们自己的自定义预设创建自定义棉绒规则!
步骤1:建立预设 (Step 1: Creating a Preset)
Presets allow you to customize elements of your Neutrino app, like ESLint rules, Babel plugins, pathing, and other app-wide global configuration. Let me first show you the code for custom linting rules and then I'll explain what's going on:
预设允许您自定义Neutrino应用程序的元素,例如ESLint规则,Babel插件,路径以及其他应用程序范围的全局配置。 首先让我向您展示自定义棉绒规则的代码,然后再说明发生了什么:
const lint = require('neutrino-lint-base');
const merge = require('deepmerge');
module.exports = neutrino => {
// Implement custom linting
lint(neutrino);
neutrino.config.module
.rule('lint')
.loader('eslint', props => merge(props, {
options: {
globals: ['describe', 'expect', 'jest', 'test', 'document', 'window', 'fetch'],
rules: {
// Don't require () for single argument arrow functions
'arrow-parens': 'off',
// Don't require trailing commas
'comma-dangle': 'off',
// Don't require file extensions on imports
'import/extensions': 'off',
// Don't mark as unresolved without extensions
'import/no-unresolved': 'off',
// Don't let ESLint tell us how to use whitespace for imports
'padded-blocks': 'off',
// Hold off on propTypes for now
'react/prop-types': 'off'
},
baseConfig: {
extends: ['airbnb-base', 'plugin:react/recommended']
}
}
}))
};
Sending neutrino
into lint
preps the Neutrino app for linting. Next we use merge
to deep merge the custom linting config with our own rules:
将neutrino
发送到lint
中将准备使用中微子应用程序。 接下来,我们使用merge
将定制的linting配置与我们自己的规则进行深度合并:
Extend
airbnb-base
linting rules with are a very popular set of ES6 guidelines通过一系列非常流行的ES6准则扩展
airbnb-base
规则- Extend recommended React.js linting guidelines扩展推荐的React.js linting指南
- Specify which globals we'll allow when linting指定掉毛时允许使用的全局变量
- Set values for very specific ESLint rules we do or don't want to enforce为我们要执行或不想执行的非常特定的ESLint规则设置值
Of course the rules I've customized above are completely my preference; you don't need to extend any existing ESLint libraries (like I did with airbnb and React) and you can enforce whichever rules you'd like.
当然,我上面自定义的规则完全是我的偏爱。 您不需要扩展任何现有的ESLint库(就像我对airbnb和React所做的那样),并且可以执行所需的任何规则。
步骤2: .eslintrc.js
(Step 2: .eslintrc.js
)
If you want to run linting from the command line at any time (in the case of CI or a post-commit hook, for example), you will need to create a .eslintrc.js
file to kick off the linting:
如果您想随时从命令行运行linting(例如,对于CI或后提交挂钩),则需要创建.eslintrc.js
文件以启动.eslintrc.js
:
const Neutrino = require('neutrino');
const pkg = require('./package.json');
const api = new Neutrino(pkg.config.presets);
module.exports = api.custom.eslintrc();
.eslintrc.js
creates a Neutrino instance with presets defined in package.json
(we'll get to that in the next section) and exposes a eslintrc()
function that runs the lint routine.
.eslintrc.js
使用在package.json
定义的预设创建一个Neutrino实例(我们将在下一部分中进行介绍),并公开运行lint例程的eslintrc()
函数。
步骤3:修改package.json
(Step 3: Modify package.json
)
With the preset created with your custom linting rules in mind, a few changes to package.json
must be made. The first is adding this custom preset file to the config.presets
array:
牢记您的自定义插入规则创建的预设,必须对package.json
进行一些更改。 首先是将此自定义预设文件添加到config.presets
数组中:
"config": {
"presets": [
"neutrino-preset-react",
"conduit-preset.js"
]
},
Next we'll need to add Neutrino's airbnb preset to our dependency list:
接下来,我们需要将Neutrino的airbnb预设添加到我们的依赖项列表中:
yarn add neutrino-preset-airbnb-base -dev
Lastly we'll add a lint
key to scripts
so that we can run linting from command line:
最后,我们将一个lint
键添加到scripts
以便我们可以从命令行运行linting:
"scripts": {
"lint": "./node_modules/eslint/bin/eslint.js --ext .js,.jsx src/ test/",
}
Now we can run the following from command line:
现在我们可以从命令行运行以下命令:
yarn lint
Also note that the custom linting rule are applied to both the manual lint
command as well as during webpack's live reload and linting routine!
还要注意,自定义的lint
规则既适用于手动lint
命令,也适用于webpack的实时重新加载和掉毛例程!
I love Neutrino because it requires minimal configuration to get up and running but custom configuration is easy when you need to. Keep an eye on Neutrino moving forward because development is shipping quickly and the community is rallying behind this amazing project!
我喜欢Neutrino,因为它需要最少的配置来启动和运行,但是自定义配置在需要时很容易。 密切注意Neutrino的前进,因为发展Swift,社区正在为这个令人惊叹的项目提供支持!
翻译自: https://davidwalsh.name/neutrino-linting
中微子超光速
http://www.taodudu.cc/news/show-3131223.html
相关文章:
- 英国物理学家冷嘲中微子超光速发现
- 一种靠加热内部空间达到超光速飞行的机器
- 一种利用电磁能产生的共振引力波实现超光速飞行的机器
- php 光速,光速 - 实验帮百科
- typescript光速入门
- 为什么宇宙会将最大速度限制在光速
- 【TJOI 2015】弦论
- C/S架构的简单文件传输系统的实现
- nyoj-56-阶乘因式分解(一)
- k8s常见报错解决
- scanf 之 %2s 与 %2d
- S3C2410驱动分析之LCD驱动
- C/S客户端渗透测试(一)客户端渗透环境配置
- 轻量级Kubernetes之k3s:2:使用docker作为容器运行环境
- k8s配置Controller Manager出错
- k8s进入容器
- S3C2410 UART驱动
- k8s跨namespace访问服务
- K8S进入容器方法
- JSP标签c:forEach实例
- H3C 交换机 OpenFlow 配置指南
- 从零开始搭建K8S--搭建K8S Ingress
- k8s node节点停机维护,pod如何迁移?
- MISRA-C 2004 规则解读(41S-60S)
- Kubernetes K8S之Pod跨namespace名称空间访问Service服务
- log4cplus日志格式输出配置
- Win11系统自带截图快捷键是什么 Win11系统自带截图工具怎么使用
- 笔记本使用计算机的快捷键是什么,笔记本电脑截屏的快捷键是什么
- android如何截屏快捷键是什么手机,安卓截屏快捷键是什么?截屏方法总结 - Android教程 - 安卓中文网...
- 三星android截屏快捷键是什么,三星截屏快捷键以及截屏方法
中微子超光速_定制中微子皮棉相关推荐
- 宇宙长城谭之二:达尔文渐变论揭开中微子超光速之谜谭
宇宙长城谭之二:达尔文渐变论揭开中微子超光速之谜谭 谭理事 达尔文是生物学家啊???!!! 有木有搞错?! 科学交叉 听说在现今信息时代,就是要搞学科交叉,才能出大学问,所以谭理事想了又想中微子超光速 ...
- 英国物理学家冷嘲中微子超光速发现
英国物理学家冷嘲中微子超光速发现 照片在英国切尔滕纳姆科学节拍摄.吉姆-阿尔-克哈里利对中微子超光速的这一惊人发现持轻蔑态度 欧洲核子研究组织(CERN)的科学家表示,他们的实验结果表明亚原子粒子中微 ...
- ftl不存在为真_科学网—世界上第一个真正的超光速实验 - 张操的博文
世界上第一个真正的超光速实验 2015年2月初,我们发表了第一篇关于交流电可以超光速实验的论文[1].近3年来,在科学网上引起了很多学者的讨论和质疑,也引发了一些理论解释的新猜想.我在这里向参加讨论的 ...
- 谭谭可以对LHC的超光速中微子视而不见吗
谭谭可以对LHC的超光速中微子视而不见吗 谭理事 本来不想开腔,因为开枪要死人! 万里之行 就我对现代科学的认识,现代科学已经发展到 "不是博士后你搞球不懂:是博士后他听球不懂" ...
- 相对论和超光速FAQ
人们所感兴趣的超光速,一般是指超光速传递能量或者信息.根据狭义相对论,这种意义下的超光速旅行和超光速通讯一般是不可能的.目前关于超光速的争论,大多数情况是某些东西的速度的确可以超过光速,但是不能用它们 ...
- 科学家发现超光速粒子 或颠覆爱因斯坦狭义相对论
欧洲研究人员发现,一些中微子能够以超过光速60纳秒的速度运行,如果这一发现被多方证实,将推翻爱因斯坦的相对论.新京报制图/帅春雷 欧洲核子研究中心外景. 欧洲核子研究中心23日宣布,他们发现一些粒子可 ...
- 霍金质疑超光速粒子发现:需要更多实验确认
本报讯 英国著名物理学家霍金(如图)对"幽灵粒子"的发现表示怀疑,他说:"目前对中微子发表评论是言之过早,还须进行更多实验及澄清工作." 对于欧洲一队科学家声称 ...
- 如何才能达到超光速?
导读:把人的思维变成"数据",变成"光一样的数据",可能吗? 铺垫文:超光速(faster-than-light, FTL或称superluminality), ...
- 量子计算 4 超光速信息传播?密度矩阵与混合态
量子计算 4 密度矩阵与混合态 1 纠缠的量子比特 2 光速的信息传播?(bushi) 3 密度矩阵(Density matrix)与混合态(Mixed state) 3.1 混合态 3.2 密度矩阵 ...
最新文章
- github如何clone别人commit的历史版本的仓库
- SVN更新文件全是最新,但缺少文件
- 久其通用数据管理平台_银保行业通用的CRM系统,为你轻松化解庞大数据难题
- linux密文解密工具,Linux之加密解密工具openssl的用法以及自建CA
- Python学习之路_day_25(面向对象之封装/多态/组合)
- 如何解决js地址栏中传递中文乱码的问题
- 深度学习花书- 4.3 基于梯度的优化方法
- 分享OUTLOOK的定时发送功能
- elment-ui的table组件多行合并
- latex插入表格:三线表格、普通表格
- python入门经典书书籍-新手Python入门经典书籍推荐
- 计算机键盘上fn键,笔记本电脑键盘上Fn键的详细介绍
- 如何搭建DHCP服务器及自动获取IP地址及相关操作
- Android:执行exec app_process启动jar失败原因
- Android 面部识别之二(调用开源面部识别算法seetaface检测)
- 放弃5k事业编选择了15k的程序员,真的值得么?
- 命令永久禁用Win10驱动程序强制签名
- Android studio 模拟器中只能输入英文 如何输入中文
- s3c6410开发板NFS挂载linux
- 软件与计算机硬件加密,51单片机程序进行软件加密和硬件解密的方法
热门文章
- 魅蓝note6救砖_魅蓝Note6线刷刷机教程 魅蓝Note6线刷包救砖刷机包下载
- python控件_python常用控件
- Oracle v$nls_parameters 和 nls_database_parameters 区别
- 《植物大战僵尸OL》中国植物曝光
- 超声波的四个特性_超声波的特性
- vue中实现拖拽调整顺序功能
- 【visual studio】符号 SymbolCache
- Vue3 之 Pinia - 状态管理
- Super-FAN论文阅读笔记
- 这两个能这样搭配?海关数据+决策人挖掘,用过的外贸人都说好!